버튼의 스타일을 커스텀화할 수 있습니다
다음은 커스텀화 하기 전에 버튼의 코드입니다.
Button(action: {
}, label: {
Text("Button")
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
})
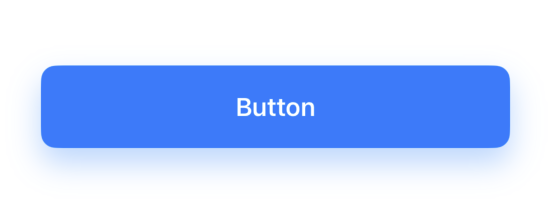
하지만 커스텀화 후에 버튼 코드는 간단합니다. 다음과 같이 ButtonStyle을 만들어주고 해당 Style을 buttonStyle에 추가합니다.
struct PressableStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
}
}
Button(action: {
}, label: {
Text("Button")
})
.buttonStyle(PressableStyle())
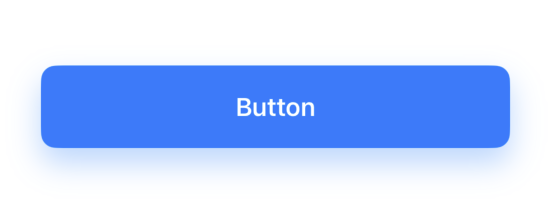
추가로 configuration의 isPressed를 사용하면 해당 스타일을 적용한 버튼이 눌렸을 때 특정 동작을 하도록 지정할수도 있습니다.
struct PressableStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
.opacity(configuration.isPressed ? 0.9 : 1.0)
//.brightness(configuration.isPressed ? 0.05 : 0)
}
}
ButtonStyle 다이나믹하게 만들어 사용하기
다음은 ButtonStyle에서 크기를 결정하는 scaleEffect의 값을 다이나믹하게 조정할 수 있도록 만든 코드입니다.
struct PressableStyle: ButtonStyle {
let scaledAmount: CGFloat
init(scaledAmount: CGFloat = 0.9) {
self.scaledAmount = scaledAmount
}
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
//.opacity(configuration.isPressed ? 0.9 : 1.0)
.brightness(configuration.isPressed ? 0.05 : 0)
.scaleEffect(configuration.isPressed ? scaledAmount : 1.0)
}
}
extension View {
func withPressableStyle(scaledAmout: CGFloat = 0.9) -> some View {
self.buttonStyle(PressableStyle())
}
}
총 코드
import SwiftUI
struct PressableStyle: ButtonStyle {
let scaledAmount: CGFloat
init(scaledAmount: CGFloat = 0.9) {
self.scaledAmount = scaledAmount
}
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
//.opacity(configuration.isPressed ? 0.9 : 1.0)
.brightness(configuration.isPressed ? 0.05 : 0)
.scaleEffect(configuration.isPressed ? scaledAmount : 1.0)
}
}
extension View {
func withPressableStyle(scaledAmout: CGFloat = 0.9) -> some View {
self.buttonStyle(PressableStyle())
}
}
struct ButtonStyleModifierExample: View {
var body: some View {
Button(action: {
}, label: {
Text("Button")
})
.withPressableStyle()
// .buttonStyle(PressableStyle(scaledAmount: 0.5))
.padding(40)
}
}
'SwiftUI' 카테고리의 다른 글
CoreBluetooth로 아두이노 불 켜기 (1) | 2024.04.07 |
---|---|
Background Threads, Queues (0) | 2024.04.07 |
Custom ViewModifiers (0) | 2024.04.07 |
@FocusState 로 키보드 다루기 (0) | 2024.04.06 |
swipeActions (0) | 2024.04.06 |
버튼의 스타일을 커스텀화할 수 있습니다
다음은 커스텀화 하기 전에 버튼의 코드입니다.
Button(action: {
}, label: {
Text("Button")
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
})
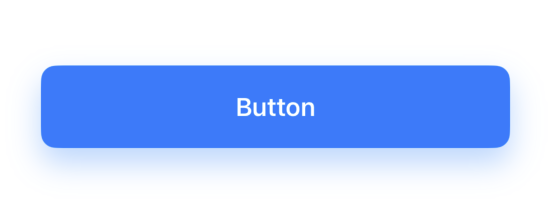
하지만 커스텀화 후에 버튼 코드는 간단합니다. 다음과 같이 ButtonStyle을 만들어주고 해당 Style을 buttonStyle에 추가합니다.
struct PressableStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
}
}
Button(action: {
}, label: {
Text("Button")
})
.buttonStyle(PressableStyle())
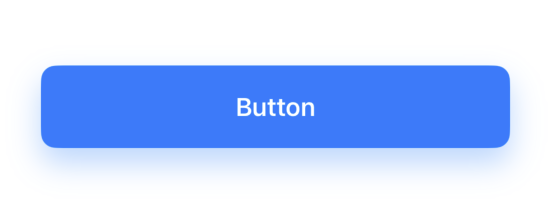
추가로 configuration의 isPressed를 사용하면 해당 스타일을 적용한 버튼이 눌렸을 때 특정 동작을 하도록 지정할수도 있습니다.
struct PressableStyle: ButtonStyle {
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
.opacity(configuration.isPressed ? 0.9 : 1.0)
//.brightness(configuration.isPressed ? 0.05 : 0)
}
}
ButtonStyle 다이나믹하게 만들어 사용하기
다음은 ButtonStyle에서 크기를 결정하는 scaleEffect의 값을 다이나믹하게 조정할 수 있도록 만든 코드입니다.
struct PressableStyle: ButtonStyle {
let scaledAmount: CGFloat
init(scaledAmount: CGFloat = 0.9) {
self.scaledAmount = scaledAmount
}
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
//.opacity(configuration.isPressed ? 0.9 : 1.0)
.brightness(configuration.isPressed ? 0.05 : 0)
.scaleEffect(configuration.isPressed ? scaledAmount : 1.0)
}
}
extension View {
func withPressableStyle(scaledAmout: CGFloat = 0.9) -> some View {
self.buttonStyle(PressableStyle())
}
}
총 코드
import SwiftUI
struct PressableStyle: ButtonStyle {
let scaledAmount: CGFloat
init(scaledAmount: CGFloat = 0.9) {
self.scaledAmount = scaledAmount
}
func makeBody(configuration: Configuration) -> some View {
configuration.label
.font(.headline)
.foregroundStyle(.white)
.frame(height: 55)
.frame(maxWidth: .infinity)
.background(Color.blue)
.clipShape(RoundedRectangle(cornerRadius: 10))
.shadow(color: Color.blue.opacity(0.3), radius: 10, x: 0.0, y: 10.0)
//.opacity(configuration.isPressed ? 0.9 : 1.0)
.brightness(configuration.isPressed ? 0.05 : 0)
.scaleEffect(configuration.isPressed ? scaledAmount : 1.0)
}
}
extension View {
func withPressableStyle(scaledAmout: CGFloat = 0.9) -> some View {
self.buttonStyle(PressableStyle())
}
}
struct ButtonStyleModifierExample: View {
var body: some View {
Button(action: {
}, label: {
Text("Button")
})
.withPressableStyle()
// .buttonStyle(PressableStyle(scaledAmount: 0.5))
.padding(40)
}
}
'SwiftUI' 카테고리의 다른 글
CoreBluetooth로 아두이노 불 켜기 (1) | 2024.04.07 |
---|---|
Background Threads, Queues (0) | 2024.04.07 |
Custom ViewModifiers (0) | 2024.04.07 |
@FocusState 로 키보드 다루기 (0) | 2024.04.06 |
swipeActions (0) | 2024.04.06 |